I want to use this example locally without docker https://fastapi-keycloak.code-specialist.com/full_example/ but this give me this error message
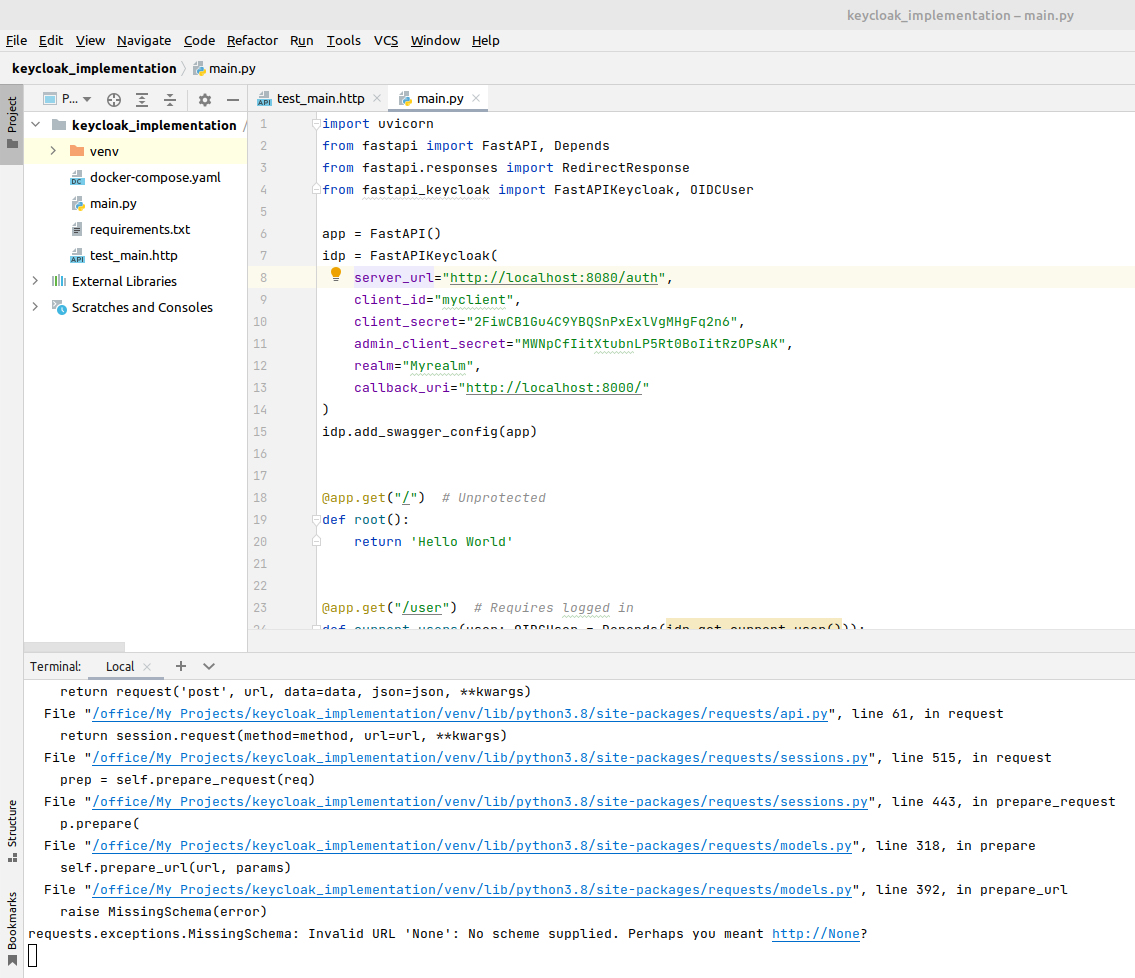
- client_secret = client > client_id > credential > Secret
- admin_client_secret = admin_cli > credential > Secret
and please specify that fastapi_keycloak will work for new keycloak version 17
The error message is
(venv) (base) sana@sana:/office/My Projects/keycloak_implementation$ uvicorn main:app --reload --port 8001
INFO: Will watch for changes in these directories: ['/office/My Projects/keycloak_implementation']
INFO: Uvicorn running on http://127.0.0.1:8001 (Press CTRL+C to quit)
INFO: Started reloader process [18160] using watchgod
Process SpawnProcess-1:
Traceback (most recent call last):
File "/usr/lib/python3.8/multiprocessing/process.py", line 315, in _bootstrap
self.run()
File "/usr/lib/python3.8/multiprocessing/process.py", line 108, in run
self._target(*self._args, **self._kwargs)
File "/office/My Projects/keycloak_implementation/venv/lib/python3.8/site-packages/uvicorn/subprocess.py", line 76, in subprocess_started
target(sockets=sockets)
File "/office/My Projects/keycloak_implementation/venv/lib/python3.8/site-packages/uvicorn/server.py", line 60, in run
return asyncio.run(self.serve(sockets=sockets))
File "/usr/lib/python3.8/asyncio/runners.py", line 44, in run
return loop.run_until_complete(main)
File "uvloop/loop.pyx", line 1501, in uvloop.loop.Loop.run_until_complete
File "/office/My Projects/keycloak_implementation/venv/lib/python3.8/site-packages/uvicorn/server.py", line 67, in serve
config.load()
File "/office/My Projects/keycloak_implementation/venv/lib/python3.8/site-packages/uvicorn/config.py", line 458, in load
self.loaded_app = import_from_string(self.app)
File "/office/My Projects/keycloak_implementation/venv/lib/python3.8/site-packages/uvicorn/importer.py", line 21, in import_from_string
module = importlib.import_module(module_str)
File "/usr/lib/python3.8/importlib/init.py", line 127, in import_module
return _bootstrap._gcd_import(name[level:], package, level)
File "", line 1014, in _gcd_import
File "", line 991, in _find_and_load
File "", line 975, in _find_and_load_unlocked
File "", line 671, in _load_unlocked
File "", line 848, in exec_module
File "", line 219, in _call_with_frames_removed
File "/office/My Projects/keycloak_implementation/./main.py", line 7, in
idp = FastAPIKeycloak(
File "/office/My Projects/keycloak_implementation/venv/lib/python3.8/site-packages/fastapi_keycloak/api.py", line 129, in init
self._get_admin_token() # Requests an admin access token on startup
File "/office/My Projects/keycloak_implementation/venv/lib/python3.8/site-packages/fastapi_keycloak/api.py", line 288, in _get_admin_token
response = requests.post(url=self.token_uri, headers=headers, data=data)
File "/office/My Projects/keycloak_implementation/venv/lib/python3.8/site-packages/requests/api.py", line 117, in post
return request('post', url, data=data, json=json, **kwargs)
File "/office/My Projects/keycloak_implementation/venv/lib/python3.8/site-packages/requests/api.py", line 61, in request
return session.request(method=method, url=url, **kwargs)
File "/office/My Projects/keycloak_implementation/venv/lib/python3.8/site-packages/requests/sessions.py", line 515, in request
prep = self.prepare_request(req)
File "/office/My Projects/keycloak_implementation/venv/lib/python3.8/site-packages/requests/sessions.py", line 443, in prepare_request
p.prepare(
File "/office/My Projects/keycloak_implementation/venv/lib/python3.8/site-packages/requests/models.py", line 318, in prepare
self.prepare_url(url, params)
File "/office/My Projects/keycloak_implementation/venv/lib/python3.8/site-packages/requests/models.py", line 392, in prepare_url
raise MissingSchema(error)
requests.exceptions.MissingSchema: Invalid URL 'None': No scheme supplied. Perhaps you meant http://None?
enhancement keycloak compatbility