Python Outlier Detection (PyOD)
Deployment & Documentation & Stats
Build Status & Coverage & Maintainability & License
PyOD is a comprehensive and scalable Python toolkit for detecting outlying objects in multivariate data. This exciting yet challenging field is commonly referred as Outlier Detection or Anomaly Detection.
PyOD includes more than 30 detection algorithms, from classical LOF (SIGMOD 2000) to the latest COPOD (ICDM 2020). Since 2017, PyOD has been successfully used in numerous academic researches and commercial products [9] [18] [29] [31]. It is also well acknowledged by the machine learning community with various dedicated posts/tutorials, including Analytics Vidhya, KDnuggets, Towards Data Science, Computer Vision News, and awesome-machine-learning.
PyOD is featured for:
- Unified APIs, detailed documentation, and interactive examples across various algorithms.
- Advanced models, including classical ones from scikit-learn, latest deep learning methods, and emerging algorithms like COPOD.
- Optimized performance with JIT and parallelization when possible, using numba and joblib.
- Compatible with both Python 2 & 3.
API Demo:
# train the COPOD detector
from pyod.models.copod import COPOD
clf = COPOD()
clf.fit(X_train)
# get outlier scores
y_train_scores = clf.decision_scores_ # raw outlier scores
y_test_scores = clf.decision_function(X_test) # outlier scores
Citing PyOD:
PyOD paper is published in JMLR (machine learning open-source software track). If you use PyOD in a scientific publication, we would appreciate citations to the following paper:
@article{zhao2019pyod, author = {Zhao, Yue and Nasrullah, Zain and Li, Zheng}, title = {PyOD: A Python Toolbox for Scalable Outlier Detection}, journal = {Journal of Machine Learning Research}, year = {2019}, volume = {20}, number = {96}, pages = {1-7}, url = {http://jmlr.org/papers/v20/19-011.html} }
or:
Zhao, Y., Nasrullah, Z. and Li, Z., 2019. PyOD: A Python Toolbox for Scalable Outlier Detection. Journal of machine learning research (JMLR), 20(96), pp.1-7.
Key Links and Resources:
Table of Contents:
- Installation
- API Cheatsheet & Reference
- Model Save & Load
- Implemented Algorithms
- Algorithm Benchmark
- Quick Start for Outlier Detection
- Quick Start for Combining Outlier Scores from Various Base Detectors
- How to Contribute
- Inclusion Criteria
Installation
It is recommended to use pip for installation. Please make sure the latest version is installed, as PyOD is updated frequently:
pip install pyod # normal install
pip install --upgrade pyod # or update if needed
pip install --pre pyod # or include pre-release version for new features
Alternatively, you could clone and run setup.py file:
git clone https://github.com/yzhao062/pyod.git
cd pyod
pip install .
Note on Python 2.7: The maintenance of Python 2.7 will be stopped by January 1, 2020 (see official announcement) To be consistent with the Python change and PyOD's dependent libraries, e.g., scikit-learn, we will stop supporting Python 2.7 in the near future (dates are still to be decided). We encourage you to use Python 3.5 or newer for the latest functions and bug fixes. More information can be found at Moving to require Python 3.
Required Dependencies:
- Python 2.7, 3.5, 3.6, or 3.7
- combo>=0.0.8
- joblib
- numpy>=1.13
- numba>=0.35
- pandas>=0.25
- scipy>=0.19.1
- scikit_learn>=0.19.1
- statsmodels
Optional Dependencies (see details below):
- keras (optional, required for AutoEncoder)
- matplotlib (optional, required for running examples)
- pandas (optional, required for running benchmark)
- tensorflow (optional, required for AutoEncoder, other backend works)
- xgboost (optional, required for XGBOD)
Warning 1: PyOD has multiple neural network based models, e.g., AutoEncoders, which are implemented in Keras. However, PyOD does NOT install keras and/or tensorflow for you. This reduces the risk of interfering with your local copies. If you want to use neural-net based models, please make sure Keras and a backend library, e.g., TensorFlow, are installed. Instructions are provided: neural-net FAQ. Similarly, models depending on xgboost, e.g., XGBOD, would NOT enforce xgboost installation by default.
Warning 2: Running examples needs matplotlib, which may throw errors in conda virtual environment on mac OS. See reasons and solutions mac_matplotlib.
Warning 3: PyOD contains multiple models that also exist in scikit-learn. However, these two libraries' API is not exactly the same--it is recommended to use only one of them for consistency but not mix the results. Refer Differences between sckit-learn and PyOD for more information.
API Cheatsheet & Reference
Full API Reference: (https://pyod.readthedocs.io/en/latest/pyod.html). API cheatsheet for all detectors:
- fit(X): Fit detector.
- decision_function(X): Predict raw anomaly score of X using the fitted detector.
- predict(X): Predict if a particular sample is an outlier or not using the fitted detector.
- predict_proba(X): Predict the probability of a sample being outlier using the fitted detector.
Key Attributes of a fitted model:
- decision_scores_: The outlier scores of the training data. The higher, the more abnormal. Outliers tend to have higher scores.
- labels_: The binary labels of the training data. 0 stands for inliers and 1 for outliers/anomalies.
Note : fit_predict() and fit_predict_score() are deprecated in V0.6.9 due to consistency issue and will be removed in V0.8.0. To get the binary labels of the training data X_train, one should call clf.fit(X_train) and use clf.labels_, instead of calling clf.predict(X_train).
Model Save & Load
PyOD takes a similar approach of sklearn regarding model persistence. See model persistence for clarification.
In short, we recommend to use joblib or pickle for saving and loading PyOD models. See "examples/save_load_model_example.py" for an example. In short, it is simple as below:
from joblib import dump, load
# save the model
dump(clf, 'clf.joblib')
# load the model
clf = load('clf.joblib')
Implemented Algorithms
PyOD toolkit consists of three major functional groups:
(i) Individual Detection Algorithms :
Type | Abbr | Algorithm | Year | Ref |
---|---|---|---|---|
Linear Model | PCA | Principal Component Analysis (the sum of weighted projected distances to the eigenvector hyperplanes) | 2003 | [27] |
Linear Model | MCD | Minimum Covariance Determinant (use the mahalanobis distances as the outlier scores) | 1999 | [10] [25] |
Linear Model | OCSVM | One-Class Support Vector Machines | 2001 | [26] |
Linear Model | LMDD | Deviation-based Outlier Detection (LMDD) | 1996 | [5] |
Proximity-Based | LOF | Local Outlier Factor | 2000 | [6] |
Proximity-Based | COF | Connectivity-Based Outlier Factor | 2002 | [28] |
Proximity-Based | CBLOF | Clustering-Based Local Outlier Factor | 2003 | [11] |
Proximity-Based | LOCI | LOCI: Fast outlier detection using the local correlation integral | 2003 | [22] |
Proximity-Based | HBOS | Histogram-based Outlier Score | 2012 | [8] |
Proximity-Based | kNN | k Nearest Neighbors (use the distance to the kth nearest neighbor as the outlier score) | 2000 | [24] |
Proximity-Based | AvgKNN | Average kNN (use the average distance to k nearest neighbors as the outlier score) | 2002 | [4] |
Proximity-Based | MedKNN | Median kNN (use the median distance to k nearest neighbors as the outlier score) | 2002 | [4] |
Proximity-Based | SOD | Subspace Outlier Detection | 2009 | [16] |
Probabilistic | ABOD | Angle-Based Outlier Detection | 2008 | [15] |
Probabilistic | COPOD | COPOD: Copula-Based Outlier Detection | 2020 | [19] |
Probabilistic | FastABOD | Fast Angle-Based Outlier Detection using approximation | 2008 | [15] |
Probabilistic | MAD | Median Absolute Deviation (MAD) | 1993 | [12] |
Probabilistic | SOS | Stochastic Outlier Selection | 2012 | [13] |
Outlier Ensembles | IForest | Isolation Forest | 2008 | [20] |
Outlier Ensembles | Feature Bagging | 2005 | [17] | |
Outlier Ensembles | LSCP | LSCP: Locally Selective Combination of Parallel Outlier Ensembles | 2019 | [31] |
Outlier Ensembles | XGBOD | Extreme Boosting Based Outlier Detection (Supervised) | 2018 | [30] |
Outlier Ensembles | LODA | Lightweight On-line Detector of Anomalies | 2016 | [23] |
Neural Networks | AutoEncoder | Fully connected AutoEncoder (use reconstruction error as the outlier score) | [1] [Ch.3] | |
Neural Networks | VAE | Variational AutoEncoder (use reconstruction error as the outlier score) | 2013 | [14] |
Neural Networks | Beta-VAE | Variational AutoEncoder (all customized loss term by varying gamma and capacity) | 2018 | [7] |
Neural Networks | SO_GAAL | Single-Objective Generative Adversarial Active Learning | 2019 | [21] |
Neural Networks | MO_GAAL | Multiple-Objective Generative Adversarial Active Learning | 2019 | [21] |
(ii) Outlier Ensembles & Outlier Detector Combination Frameworks:
Type | Abbr | Algorithm | Year | Ref |
---|---|---|---|---|
Outlier Ensembles | Feature Bagging | 2005 | [17] | |
Outlier Ensembles | LSCP | LSCP: Locally Selective Combination of Parallel Outlier Ensembles | 2019 | [31] |
Outlier Ensembles | XGBOD | Extreme Boosting Based Outlier Detection (Supervised) | 2018 | [30] |
Outlier Ensembles | LODA | Lightweight On-line Detector of Anomalies | 2016 | [23] |
Combination | Average | Simple combination by averaging the scores | 2015 | [2] |
Combination | Weighted Average | Simple combination by averaging the scores with detector weights | 2015 | [2] |
Combination | Maximization | Simple combination by taking the maximum scores | 2015 | [2] |
Combination | AOM | Average of Maximum | 2015 | [2] |
Combination | MOA | Maximization of Average | 2015 | [2] |
Combination | Median | Simple combination by taking the median of the scores | 2015 | [2] |
Combination | majority Vote | Simple combination by taking the majority vote of the labels (weights can be used) | 2015 | [2] |
(iii) Utility Functions:
Type | Name | Function | Documentation |
---|---|---|---|
Data | generate_data | Synthesized data generation; normal data is generated by a multivariate Gaussian and outliers are generated by a uniform distribution | generate_data |
Data | generate_data_clusters | Synthesized data generation in clusters; more complex data patterns can be created with multiple clusters | generate_data_clusters |
Stat | wpearsonr | Calculate the weighted Pearson correlation of two samples | wpearsonr |
Utility | get_label_n | Turn raw outlier scores into binary labels by assign 1 to top n outlier scores | get_label_n |
Utility | precision_n_scores | calculate precision @ rank n | precision_n_scores |
Algorithm Benchmark
The comparison among of implemented models is made available below (Figure, compare_all_models.py, Interactive Jupyter Notebooks). For Jupyter Notebooks, please navigate to "/notebooks/Compare All Models.ipynb".
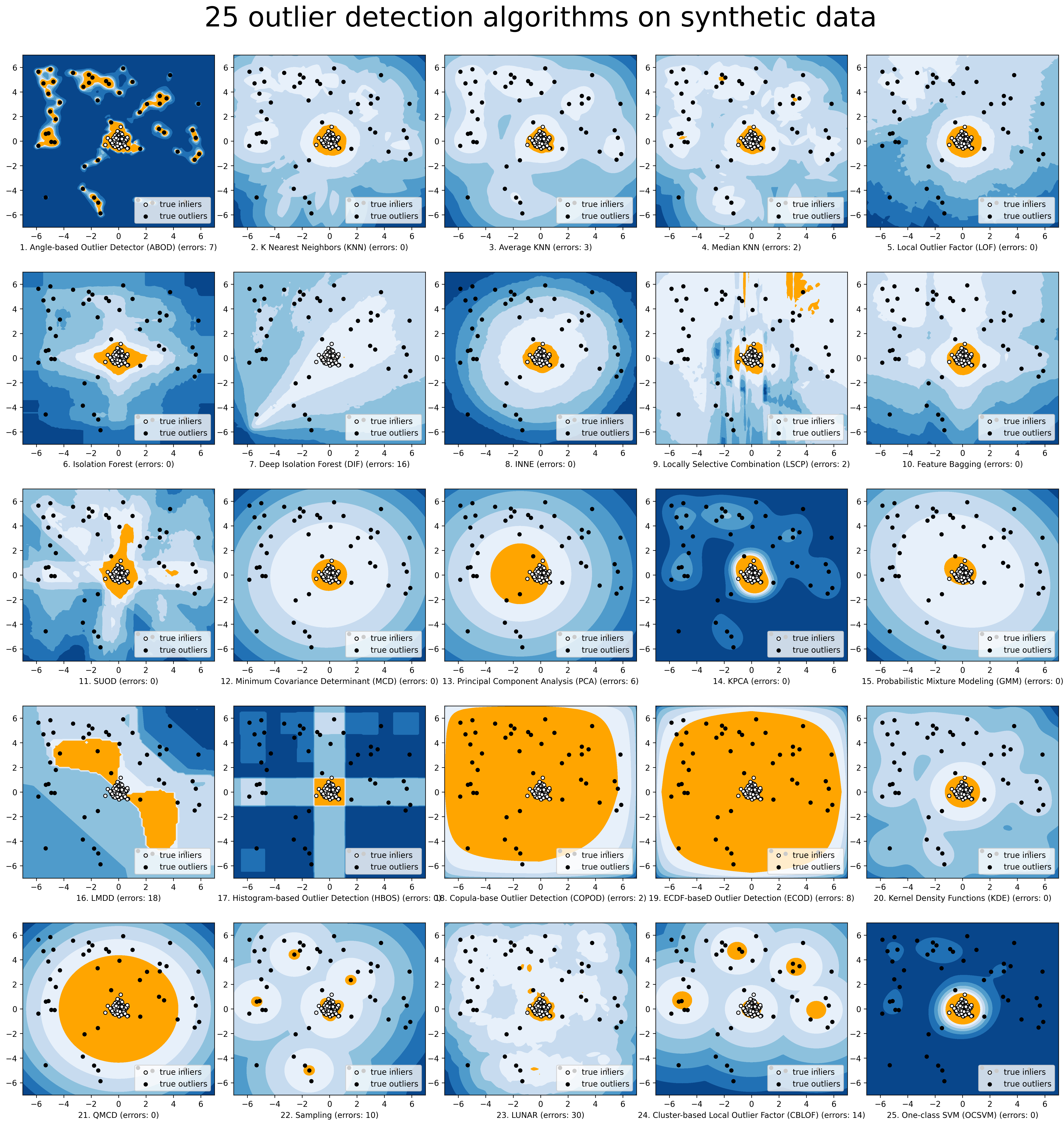
A benchmark is supplied for select algorithms to provide an overview of the implemented models. In total, 17 benchmark datasets are used for comparison, which can be downloaded at ODDS.
For each dataset, it is first split into 60% for training and 40% for testing. All experiments are repeated 10 times independently with random splits. The mean of 10 trials is regarded as the final result. Three evaluation metrics are provided:
- The area under receiver operating characteristic (ROC) curve
- Precision @ rank n (P@N)
- Execution time
Check the latest benchmark. You could replicate this process by running benchmark.py.
Quick Start for Outlier Detection
PyOD has been well acknowledged by the machine learning community with a few featured posts and tutorials.
Analytics Vidhya: An Awesome Tutorial to Learn Outlier Detection in Python using PyOD Library
KDnuggets: Intuitive Visualization of Outlier Detection Methods, An Overview of Outlier Detection Methods from PyOD
Towards Data Science: Anomaly Detection for Dummies
Computer Vision News (March 2019): Python Open Source Toolbox for Outlier Detection
"examples/knn_example.py" demonstrates the basic API of using kNN detector. It is noted that the API across all other algorithms are consistent/similar.
More detailed instructions for running examples can be found in examples directory.
Initialize a kNN detector, fit the model, and make the prediction.
from pyod.models.knn import KNN # kNN detector # train kNN detector clf_name = 'KNN' clf = KNN() clf.fit(X_train) # get the prediction label and outlier scores of the training data y_train_pred = clf.labels_ # binary labels (0: inliers, 1: outliers) y_train_scores = clf.decision_scores_ # raw outlier scores # get the prediction on the test data y_test_pred = clf.predict(X_test) # outlier labels (0 or 1) y_test_scores = clf.decision_function(X_test) # outlier scores
Evaluate the prediction by ROC and Precision @ Rank n (p@n).
from pyod.utils.data import evaluate_print # evaluate and print the results print("\nOn Training Data:") evaluate_print(clf_name, y_train, y_train_scores) print("\nOn Test Data:") evaluate_print(clf_name, y_test, y_test_scores)
See a sample output & visualization.
On Training Data: KNN ROC:1.0, precision @ rank n:1.0 On Test Data: KNN ROC:0.9989, precision @ rank n:0.9
visualize(clf_name, X_train, y_train, X_test, y_test, y_train_pred, y_test_pred, show_figure=True, save_figure=False)
Visualization (knn_figure):
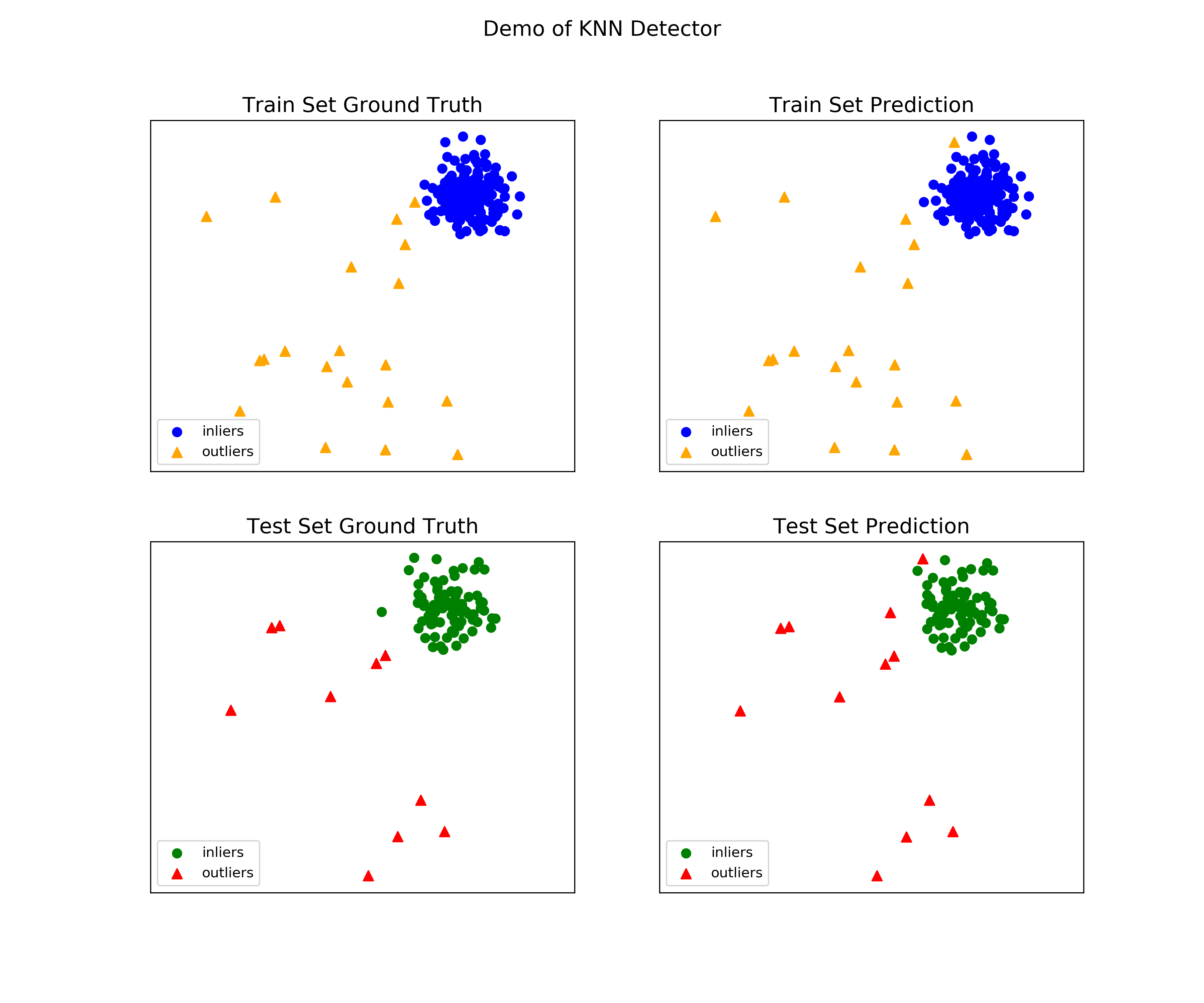
Quick Start for Combining Outlier Scores from Various Base Detectors
Outlier detection often suffers from model instability due to its unsupervised nature. Thus, it is recommended to combine various detector outputs, e.g., by averaging, to improve its robustness. Detector combination is a subfield of outlier ensembles; refer [3] for more information.
Four score combination mechanisms are shown in this demo:
- Average: average scores of all detectors.
- maximization: maximum score across all detectors.
- Average of Maximum (AOM): divide base detectors into subgroups and take the maximum score for each subgroup. The final score is the average of all subgroup scores.
- Maximum of Average (MOA): divide base detectors into subgroups and take the average score for each subgroup. The final score is the maximum of all subgroup scores.
"examples/comb_example.py" illustrates the API for combining the output of multiple base detectors (comb_example.py, Jupyter Notebooks). For Jupyter Notebooks, please navigate to "/notebooks/Model Combination.ipynb"
Import models and generate sample data.
from pyod.models.knn import KNN from pyod.models.combination import aom, moa, average, maximization from pyod.utils.data import generate_data X, y = generate_data(train_only=True) # load data
First initialize 20 kNN outlier detectors with different k (10 to 200), and get the outlier scores.
# initialize 20 base detectors for combination k_list = [10, 20, 30, 40, 50, 60, 70, 80, 90, 100, 110, 120, 130, 140, 150, 160, 170, 180, 190, 200] train_scores = np.zeros([X_train.shape[0], n_clf]) test_scores = np.zeros([X_test.shape[0], n_clf]) for i in range(n_clf): k = k_list[i] clf = KNN(n_neighbors=k, method='largest') clf.fit(X_train_norm) train_scores[:, i] = clf.decision_scores_ test_scores[:, i] = clf.decision_function(X_test_norm)
Then the output scores are standardized into zero mean and unit variance before combination. This step is crucial to adjust the detector outputs to the same scale.
from pyod.utils.utility import standardizer train_scores_norm, test_scores_norm = standardizer(train_scores, test_scores)
Then four different combination algorithms are applied as described above.
comb_by_average = average(test_scores_norm) comb_by_maximization = maximization(test_scores_norm) comb_by_aom = aom(test_scores_norm, 5) # 5 groups comb_by_moa = moa(test_scores_norm, 5)) # 5 groups
Finally, all four combination methods are evaluated with ROC and Precision @ Rank n.
Combining 20 kNN detectors Combination by Average ROC:0.9194, precision @ rank n:0.4531 Combination by Maximization ROC:0.9198, precision @ rank n:0.4688 Combination by AOM ROC:0.9257, precision @ rank n:0.4844 Combination by MOA ROC:0.9263, precision @ rank n:0.4688
How to Contribute
You are welcome to contribute to this exciting project:
- Please first check Issue lists for "help wanted" tag and comment the one you are interested. We will assign the issue to you.
- Fork the master branch and add your improvement/modification/fix.
- Create a pull request to development branch and follow the pull request template PR template
- Automatic tests will be triggered. Make sure all tests are passed. Please make sure all added modules are accompanied with proper test functions.
To make sure the code has the same style and standard, please refer to abod.py, hbos.py, or feature_bagging.py for example.
You are also welcome to share your ideas by opening an issue or dropping me an email at [email protected] :)
Inclusion Criteria
Similarly to scikit-learn, We mainly consider well-established algorithms for inclusion. A rule of thumb is at least two years since publication, 50+ citations, and usefulness.
However, we encourage the author(s) of newly proposed models to share and add your implementation into PyOD for boosting ML accessibility and reproducibility. This exception only applies if you could commit to the maintenance of your model for at least two year period.
Reference
[1] | Aggarwal, C.C., 2015. Outlier analysis. In Data mining (pp. 237-263). Springer, Cham. |
[2] | (1, 2, 3, 4, 5, 6, 7) Aggarwal, C.C. and Sathe, S., 2015. Theoretical foundations and algorithms for outlier ensembles.ACM SIGKDD Explorations Newsletter, 17(1), pp.24-47. |
[3] | Aggarwal, C.C. and Sathe, S., 2017. Outlier ensembles: An introduction. Springer. |
[4] | (1, 2) Angiulli, F. and Pizzuti, C., 2002, August. Fast outlier detection in high dimensional spaces. In European Conference on Principles of Data Mining and Knowledge Discovery pp. 15-27. |
[5] | Arning, A., Agrawal, R. and Raghavan, P., 1996, August. A Linear Method for Deviation Detection in Large Databases. In KDD (Vol. 1141, No. 50, pp. 972-981). |
[6] | Breunig, M.M., Kriegel, H.P., Ng, R.T. and Sander, J., 2000, May. LOF: identifying density-based local outliers. ACM Sigmod Record, 29(2), pp. 93-104. |
[7] | Burgess, Christopher P., et al. "Understanding disentangling in beta-VAE." arXiv preprint arXiv:1804.03599 (2018). |
[8] | Goldstein, M. and Dengel, A., 2012. Histogram-based outlier score (hbos): A fast unsupervised anomaly detection algorithm. In KI-2012: Poster and Demo Track, pp.59-63. |
[9] | Gopalan, P., Sharan, V. and Wieder, U., 2019. PIDForest: Anomaly Detection via Partial Identification. In Advances in Neural Information Processing Systems, pp. 15783-15793. |
[10] | Hardin, J. and Rocke, D.M., 2004. Outlier detection in the multiple cluster setting using the minimum covariance determinant estimator. Computational Statistics & Data Analysis, 44(4), pp.625-638. |
[11] | He, Z., Xu, X. and Deng, S., 2003. Discovering cluster-based local outliers. Pattern Recognition Letters, 24(9-10), pp.1641-1650. |
[12] | Iglewicz, B. and Hoaglin, D.C., 1993. How to detect and handle outliers (Vol. 16). Asq Press. |
[13] | Janssens, J.H.M., Huszár, F., Postma, E.O. and van den Herik, H.J., 2012. Stochastic outlier selection. Technical report TiCC TR 2012-001, Tilburg University, Tilburg Center for Cognition and Communication, Tilburg, The Netherlands. |
[14] | Kingma, D.P. and Welling, M., 2013. Auto-encoding variational bayes. arXiv preprint arXiv:1312.6114. |
[15] | (1, 2) Kriegel, H.P. and Zimek, A., 2008, August. Angle-based outlier detection in high-dimensional data. In KDD '08, pp. 444-452. ACM. |
[16] | Kriegel, H.P., Kröger, P., Schubert, E. and Zimek, A., 2009, April. Outlier detection in axis-parallel subspaces of high dimensional data. In Pacific-Asia Conference on Knowledge Discovery and Data Mining, pp. 831-838. Springer, Berlin, Heidelberg. |
[17] | (1, 2) Lazarevic, A. and Kumar, V., 2005, August. Feature bagging for outlier detection. In KDD '05. 2005. |
[18] | Li, D., Chen, D., Jin, B., Shi, L., Goh, J. and Ng, S.K., 2019, September. MAD-GAN: Multivariate anomaly detection for time series data with generative adversarial networks. In International Conference on Artificial Neural Networks (pp. 703-716). Springer, Cham. |
[19] | Li, Z., Zhao, Y., Botta, N., Ionescu, C. and Hu, X. COPOD: Copula-Based Outlier Detection. IEEE International Conference on Data Mining (ICDM), 2020. |
[20] | Liu, F.T., Ting, K.M. and Zhou, Z.H., 2008, December. Isolation forest. In International Conference on Data Mining, pp. 413-422. IEEE. |
[21] | (1, 2) Liu, Y., Li, Z., Zhou, C., Jiang, Y., Sun, J., Wang, M. and He, X., 2019. Generative adversarial active learning for unsupervised outlier detection. IEEE Transactions on Knowledge and Data Engineering. |
[22] | Papadimitriou, S., Kitagawa, H., Gibbons, P.B. and Faloutsos, C., 2003, March. LOCI: Fast outlier detection using the local correlation integral. In ICDE '03, pp. 315-326. IEEE. |
[23] | (1, 2) Pevný, T., 2016. Loda: Lightweight on-line detector of anomalies. Machine Learning, 102(2), pp.275-304. |
[24] | Ramaswamy, S., Rastogi, R. and Shim, K., 2000, May. Efficient algorithms for mining outliers from large data sets. ACM Sigmod Record, 29(2), pp. 427-438. |
[25] | Rousseeuw, P.J. and Driessen, K.V., 1999. A fast algorithm for the minimum covariance determinant estimator. Technometrics, 41(3), pp.212-223. |
[26] | Scholkopf, B., Platt, J.C., Shawe-Taylor, J., Smola, A.J. and Williamson, R.C., 2001. Estimating the support of a high-dimensional distribution. Neural Computation, 13(7), pp.1443-1471. |
[27] | Shyu, M.L., Chen, S.C., Sarinnapakorn, K. and Chang, L., 2003. A novel anomaly detection scheme based on principal component classifier. MIAMI UNIV CORAL GABLES FL DEPT OF ELECTRICAL AND COMPUTER ENGINEERING. |
[28] | Tang, J., Chen, Z., Fu, A.W.C. and Cheung, D.W., 2002, May. Enhancing effectiveness of outlier detections for low density patterns. In Pacific-Asia Conference on Knowledge Discovery and Data Mining, pp. 535-548. Springer, Berlin, Heidelberg. |
[29] | Wang, X., Du, Y., Lin, S., Cui, P., Shen, Y. and Yang, Y., 2019. adVAE: A self-adversarial variational autoencoder with Gaussian anomaly prior knowledge for anomaly detection. Knowledge-Based Systems. |
[30] | (1, 2) Zhao, Y. and Hryniewicki, M.K. XGBOD: Improving Supervised Outlier Detection with Unsupervised Representation Learning. IEEE International Joint Conference on Neural Networks, 2018. |
[31] | (1, 2, 3) Zhao, Y., Nasrullah, Z., Hryniewicki, M.K. and Li, Z., 2019, May. LSCP: Locally selective combination in parallel outlier ensembles. In Proceedings of the 2019 SIAM International Conference on Data Mining (SDM), pp. 585-593. Society for Industrial and Applied Mathematics. |