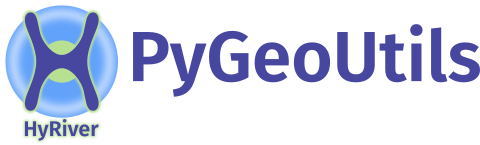
Package | Description | Status |
---|---|---|
PyNHD | Navigate and subset NHDPlus (MR and HR) using web services | |
Py3DEP | Access topographic data through National Map's 3DEP web service | |
PyGeoHydro | Access NWIS, NID, WQP, HCDN 2009, NLCD, and SSEBop databases | |
PyDaymet | Access Daymet for daily climate data both single pixel and gridded | |
AsyncRetriever | High-level API for asynchronous requests with persistent caching | |
PyGeoOGC | Send queries to any ArcGIS RESTful-, WMS-, and WFS-based services | |
PyGeoUtils | Convert responses from PyGeoOGC's supported web services to datasets |
PyGeoUtils: Utilities for (Geo)JSON and (Geo)TIFF Conversion
Features
PyGeoUtils is a part of HyRiver software stack that is designed to aid in watershed analysis through web services. This package provides utilities for manipulating (Geo)JSON and (Geo)TIFF responses from web services. These utilities are:
json2geodf
: For converting (Geo)JSON objects to GeoPandas dataframe.arcgis2geojson
: For converting ESRIGeoJSON to the standard GeoJSON format.gtiff2xarray
: For converting (Geo)TIFF objects to xarray. datasets.xarray2geodf
: For convertingxarray.DataArray
to ageopandas.GeoDataFrame
, i.e., vectorization.xarray_geomask
: For masking axarray.Dataset
orxarray.DataArray
using a polygon.
All these functions handle all necessary CRS transformations.
You can find some example notebooks here.
Please note that since this project is in early development stages, while the provided functionalities should be stable, changes in APIs are possible in new releases. But we appreciate it if you give this project a try and provide feedback. Contributions are most welcome.
Moreover, requests for additional functionalities can be submitted via issue tracker.
Installation
You can install PyGeoUtils using pip
after installing libgdal
on your system (for example, in Ubuntu run sudo apt install libgdal-dev
). Moreover, PyGeoUtils has an optional dependency for using persistent caching, requests-cache
. We highly recommend to install this package as it can significantly speedup send/receive queries. You don't have to change anything in your code, since PyGeoUtils under-the-hood looks for requests-cache
and if available, it will automatically use persistent caching:
$ pip install pygeoutils
Alternatively, PyGeoUtils can be installed from the conda-forge
repository using Conda:
$ conda install -c conda-forge pygeoutils
Quick start
To demonstrate capabilities of PyGeoUtils let's use PyGeoOGC to access National Wetlands Inventory from WMS, and FEMA National Flood Hazard via WFS, then convert the output to xarray.Dataset
and GeoDataFrame
, respectively.
import pygeoutils as geoutils
from pygeoogc import WFS, WMS, ServiceURL
from shapely.geometry import Polygon
geometry = Polygon(
[
[-118.72, 34.118],
[-118.31, 34.118],
[-118.31, 34.518],
[-118.72, 34.518],
[-118.72, 34.118],
]
)
crs = "epsg:4326"
wms = WMS(
ServiceURL().wms.mrlc,
layers="NLCD_2011_Tree_Canopy_L48",
outformat="image/geotiff",
crs=crs,
)
r_dict = wms.getmap_bybox(
geometry.bounds,
1e3,
box_crs=crs,
)
canopy = geoutils.gtiff2xarray(r_dict, geometry, crs)
mask = canopy > 60
canopy_gdf = geoutils.xarray2geodf(canopy, "float32", mask)
url_wfs = "https://hazards.fema.gov/gis/nfhl/services/public/NFHL/MapServer/WFSServer"
wfs = WFS(
url_wfs,
layer="public_NFHL:Base_Flood_Elevations",
outformat="esrigeojson",
crs="epsg:4269",
)
r = wfs.getfeature_bybox(geometry.bounds, box_crs=crs)
flood = geoutils.json2geodf(r.json(), "epsg:4269", crs)
Contributing
Contributions are very welcomed. Please read CONTRIBUTING.rst file for instructions.